Saturday, September 20, 2008
Issue with maven and cobertura integration
I have done following modificaitons in pom.xml file:
a) Added plug-in for cobertura
<build>
...
<plugins>
...
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>cobertura-maven-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>clean</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
b) Reporting
<reporting>
<plugins>
...
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>cobertura-maven-plugin</artifactId>
</plugin>
</plugins>
</reporting>
When I ran mvn cobertura:cobertura, I got the following error:
[INFO] Nothing to compile - all classes are up to date [INFO] [cobertura:instrument] [ERROR] java.lang.UnsupportedClassVersionError: net/sourceforge/cobertura/instru ment/Main (Unsupported major.minor version 49.0) at java.lang.ClassLoader.defineClass0(Native Method) at java.lang.ClassLoader.defineClass(Unknown Source) at java.security.SecureClassLoader.defineClass(Unknown Source) at java.net.URLClassLoader.defineClass(Unknown Source) at java.net.URLClassLoader.access$100(Unknown Source) at java.net.URLClassLoader$1.run(Unknown Source) at java.security.AccessController.doPrivileged(Native Method) at java.net.URLClassLoader.findClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClassInternal(Unknown Source)
By looking at this I came to a conclusion that this incompatible class version issue.
When i ran mvn –version command, it gave me following info. Evrything looks fine as expected.
Maven version: 2.0.9
Java version: 1.5.0_15 OS name: "windows xp" version: "5.1" arch: "x86" Family: "windows"
I should not see above exception as I am using right version of JDK (1.5.0_15). I checked JAVA_HOME and even JRE_HOME env variable and they pointed to right location.
When I executed java –version command, I see following results
java version "1.4.2_11" Java(TM) 2 Runtime Environment, Standard Edition (build 1.4.2_11-b06) Java HotSpot(TM) Client VM (build 1.4.2_11-b06, mixed mode)
Hmmmm…
I still didn’t believe that’s the root cause of problem. I gave it a
try by uninstalling JRE 1.4.2 and installed JRE 1.5 and everything started working fine.
I believe(though I am not a maven guru) that MAVEN is not making use of environment variable (here JAVA_HOME) to execute the plug in. I have spent almost 3 hours of my time and i thought this might be helpful for people like me.
Saturday, February 09, 2008
On Struts 2
http://www.infoq.com/articles/converting-struts-2-part1
http://www.infoq.com/articles/migrating-struts-2-part2
Summary of points:
- Dispatcher has been changed from servlet to filter
- Action class is not singleton. So no thread-safety issues for Action class
- Action class can be a simple POJO with a signature public String methodName
- No tight coupling to container in Action class code.
- Injected dependencies with DI/IoC
- Concept of Interceptors
- Simplified Unit testing of action classes
Tuesday, March 27, 2007
Spring's IoC
Sunday, March 25, 2007
JSF Lifecycle
JSF Lifecycle Phases
1. Restore View
- If the request is first visit of page, builds the view of the page, wire event handlers and validator to each component in view and saves view in FacesContext
- If the action is post back, it restores the view which had already been created
2. Apply request values
- Recursively calls decode() on all the components in tree by extracting new values from request
- In case of conversion failure, stacks up error messages in faces context
- If there are any events queued up, notifies the interested listeners
- If any of the components have immediate attribute set to 'true', conversion, validation and events associated with component are done in this phase
- If the redirection to another web app or non-JSF page required, responseComplete() called on FacesContext
- If the validators or listeners call FacesContext’s renderResponse method, the lifecycle skips to render response phase.
3. Process Validations
- Registered validators on components triggered and error messages would be stacked into faces context if there are any validation issues
- If there are any events queued up, notifies the interested listeners
- If the redirection to another web app or non-JSF page required, responseComplete() called on FacesContext
- If the validators or listeners call FacesContext.renderResponse method, the lifecycle skips to render response phase.
4. Update model values phase
- Copies the values to backed bean properties
- In case of conversion issues, lifecycle advances to render response phase
- If the updateModels or listerners call FacesContext.renderResponse method, the lifecycle skips to render response phase.
- If there are any events queued up, notifies the interested listeners.
- If the redirection to another web app or non-JSF app required, responseComplete() called on FacesContext
- Invoke Application Phase
5. Handles the application level events
- If the redirection to another web app or non-JSF app required, responseComplete() called on FacesContext
6. Render Response Phase
- Renders the response
- If there are any validation errors, renders the error messages on page if there are messages tags.
Saturday, March 24, 2007
Coding with JSF is more OO
With JSF, managed beans concept is removing the extra class and giving flexibility to write the data and behavior in same class.
Sunday, September 24, 2006
Java Server Faces
I was looking into Apache Shale today. This framework is built on all JSF features. JSF can be used even with struts but it might introduce lot of redundant code. Struts design is with MVC2 pattern, which was invented almost 5 years back, and I think its time to move on to Shale framework for next generation of web application development for more flexibility.
Saturday, September 23, 2006
Points on Architecture
Why Draw an SSD?
An interesting and useful question in software design is this: What events are coming in to our system? Why? Because we have to design the software to handle these events (from the mouse, keyboard, another system, …) and execute a response.
Basically, a software system reacts to three things:
1) external events from actors (humans or computers),
2) timer events, and
3) faults or exceptions (which are often from external sources).
What is the Relationship Between SSDs and Use Cases?
An SSD shows system events for one scenario of a use case, therefore it is generated from inspection of a use case
What is the Logical Architecture? And Layers?
The logical architecture is the large-scale organization of the software classes into packages (or namespaces), subsystems, and layers. It's called the logical architecture because there's no decision about how these elements are deployed across different operating system processes or across physical computers in a network (these latter decisions are part of the deployment architecture).
A layer is a very coarse-grained grouping of classes, packages, or subsystems that has cohesive responsibility for a major aspect of the system. Also, layers are organized such that "higher" layers (such as the UI layer) call upon services of "lower" layers, but not normally vice versa.
Typically layers in an OO system include:
User Interface.
Application Logic and Domain Objects— software objects representing domain concepts (for example, a software class Sale) that fulfill application requirements, such as calculating a sale total.
Technical Services— general purpose objects and subsystems that provide supporting technical services, such as interfacing with a database or error logging. These services are usually application-independent and reusable across several systems.
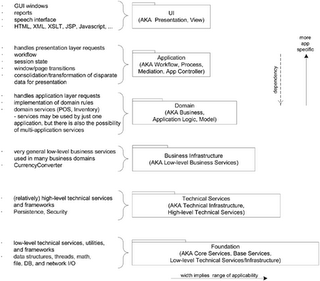